ReactJS is an open-source JavaScript library developed by Facebook’s Jordan Walke to facilitate the creation of user interfaces for both web and mobile systems. It first saw use in 2011 on Facebook’s newsfeed, followed by Instagram in 2012, and was made publicly available in 2013. React excels in applications with rapidly changing data, allowing for efficient updates to specific parts of the user interface without needing to reload the entire page. This feature significantly enhances the user experience by improving speed and responsiveness.
ReactJS revolutionizes user interface development with its efficient data handling, enabling seamless updates for enhanced speed and responsiveness.
What is the Purpose of ReactJS?
JavaScript has experienced a surge in popularity, becoming the go-to language for handling an application’s views, whether for web or mobile platforms, as well as for reusing UI components. ReactJS enables developers to construct web applications capable of dynamically updating data without requiring a page reload. Its ease of use and speed make it a preferred choice, functioning akin to a view in an MVC template or as a collection of JavaScript libraries or frameworks.
ReactJS’s primary objective is to optimize the loading speed of user interfaces in applications. It achieves this through the use of a virtual DOM, a JavaScript object that enhances the performance of ReactJS applications compared to those using the real DOM. ReactJS can be utilized on both the server and client sides, in conjunction with other frameworks, employing data patterns and components to enhance code readability and facilitate app maintenance.
Is React Front-End or Back-End?
ReactJS is often a subject of debate regarding whether it is a framework or a library and whether it pertains to front-end or back-end web development. React leverages JavaScript to create interactive user interfaces primarily for single-page applications, handling the view layer and enabling the creation of modular UI components.
ReactJS predominantly operates on the front-end, significantly impacting front-end development with its widespread adoption. This enables ReactJS developers to craft versatile web applications with dynamic data updates that occur seamlessly without page reloads. Post-deployment, ReactJS continues to enhance applications by boosting speed and introducing new features. Its standout features include speed, flexibility, usability, performance, and the ability to create reusable components, distinguishing it from other front-end development frameworks.
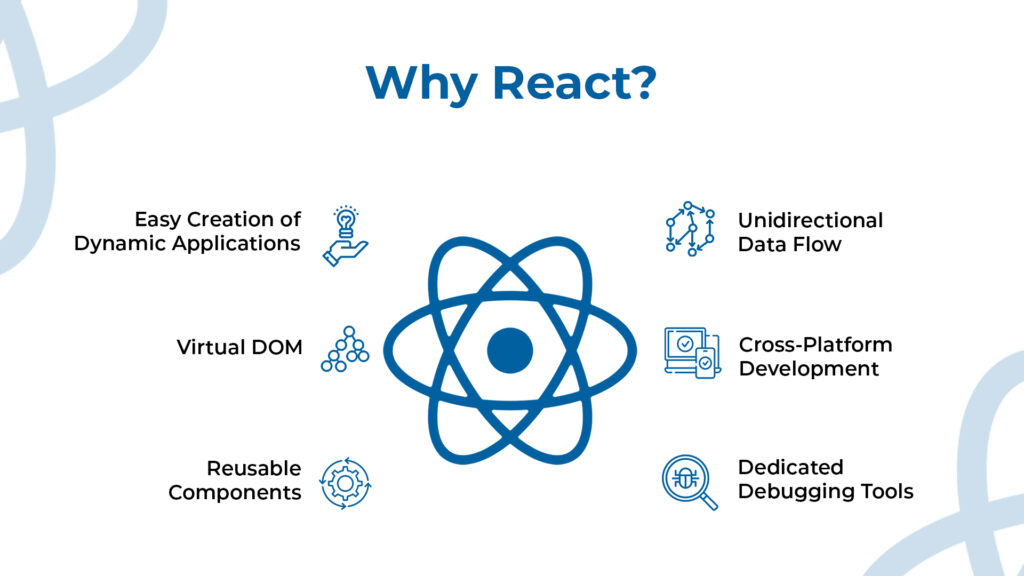
Main Features of ReactJS
ReactJS, being one of the most prominent JavaScript frameworks, offers excessive features that make it highly beneficial for web developers. These features contribute significantly to its popularity and widespread adoption in the front-end ecosystem. Let’s delve into the main features of ReactJS:
JSX (JavaScript Syntax Extension): JSX, which stands for JavaScript XML, is a syntax extension utilized in ReactJS. It allows developers to write code using a syntax that resembles XML or HTML within JavaScript. JSX is then transformed into standard JavaScript function calls by React. While using JSX is not mandatory in React, it is highly recommended due to its ability to seamlessly integrate HTML-like syntax with JavaScript code, making React development more intuitive and efficient.
Virtual DOM: The Document Object Model (DOM) is pivotal in web development as it represents the structure of a web page. Traditionally, JavaScript frameworks update the entire DOM when changes occur, resulting in performance inefficiencies. React, however, employs a Virtual DOM, which is a lightweight copy of the real DOM. When modifications are made to a React application, the Virtual DOM is updated first, and then a comparison is made with the real DOM to identify the differences. Subsequently, only the parts of the DOM that have changed are updated, leading to enhanced performance and a smoother user experience.
One-way Data Binding: React follows a one-way data binding approach, where data flows only in one direction, typically from parent components to child components. This ensures a clear and predictable data flow within the application. While child components can receive data from parent components through props, they cannot directly modify the data of their parent components. This unidirectional data flow enhances modularity and facilitates efficient data management within React applications.
Declarative: React emphasizes a declarative programming paradigm, where developers describe the desired state of the user interface, and React takes care of updating and rendering the components accordingly. This approach simplifies the development process, as developers can focus on defining the desired outcomes rather than worrying about the underlying implementation details. React’s declarative nature results in more concise and maintainable code, reducing development time and costs.
React Native: React Native extends the capabilities of React beyond web development to mobile app development. It enables developers to build native mobile applications using JavaScript and React principles. Unlike traditional hybrid frameworks, React Native utilizes native components, providing a more seamless and performant user experience. Developers familiar with React can leverage their skills to create cross-platform mobile applications with React Native, further enhancing productivity and code reusability.
Component-Based: ReactJS adopts a component-based architecture, where the user interface is composed of reusable and independent components. Each component encapsulates its own logic, state, and rendering, promoting modularity and reusability. This component-based approach simplifies the development process, as developers can break down complex user interfaces into smaller, manageable components. Additionally, React’s ability to define components using JavaScript enables the integration of rich data and logic within the components, while keeping the DOM manipulation separate.
What is JSX, the Basic Syntax Of ReactJS?
JSX, or JavaScript XML, is a syntax extension in ReactJS that allows developers to write HTML-like code directly within JavaScript. This feature simplifies the process of creating templates in React by enabling the integration of markup and logic in a single file. JSX is not a separate templating language; instead, it leverages the full power of JavaScript, making it a versatile and powerful tool for building user interfaces.
One of the key advantages of JSX is its performance optimization. JSX is transformed into standard JavaScript code during compilation, which allows for efficient rendering and updates. By combining markup and logic within JSX, React components become more cohesive and easier to manage. This approach contrasts with traditional methods of separating markup and logic into distinct files.
Components of ReactJS
In ReactJS, components serve as the building blocks of user interfaces, allowing developers to create modular and reusable pieces of UI. These components encapsulate both the visual elements and the logic needed to interact with them, promoting a more organized and maintainable codebase.
There are two main types of components in ReactJS: class components and functional components.
Class Components:
These are JavaScript classes that extend from React.Component. They have access to React’s lifecycle methods and can maintain their own internal state. Lifecycle methods are special functions that get called automatically at specific points in a component’s life, such as when it’s first rendered to the screen or when it’s removed from the screen. Class components are more powerful and are used when a component needs to manage state or handle lifecycle events.
Example:
class Car extends React.Component { render() { return <h2>Hi, I am a Car!</h2> } }
Functional Components:
These are simpler JavaScript functions that accept props (short for properties) as arguments and return JSX (JavaScript XML) to describe the UI. Functional components are primarily used for presenting UI elements and do not have their own internal state or lifecycle methods. They are lightweight and easier to understand, making them a preferred choice for simpler UI elements.
Example:
function Car() { return <h2>Hi, I am a Car!</h2> }
Rendering a Component:
To use a component in your React application, you simply include it as if it were a regular HTML element. You can use the component’s name as a custom HTML tag. React takes care of rendering the component and updating it when necessary.
Here’s how you render the Car component:
ReactDOM.render(<Car />, document.getElementById('root'));
This code snippet tells React to render the Car component inside the HTML element with the ID root. React then replaces the content of the root element with the output of the Car component’s render function, which in this case is the <h2> element displaying “Hi, I am a Car!”.
Component Interaction
In React applications, components often need to communicate with each other to create dynamic and interactive user interfaces. Understanding how components interact is crucial for building complex and efficient applications. React provides several mechanisms for component interaction, each serving specific use cases. Let’s explore these interaction patterns in detail:
1. Parent-to-Child Communication with Props:
Props, short for properties, are the primary means of communication between parent and child components in React. Props are passed from parent components to child components as attributes and are accessible within the child component as properties. They enable the parent component to pass data down to its children.
return <div> <AppChild name="Matt" /> </div> } function AppChild(props){ return <span> My name is {props.name} </span> } ReactDOM.render(<App />, document.getElementById('app'));
In this example, the ParentComponent passes the name prop to the ChildComponent, which renders it.
2. Child-to-Parent Communication with Callback Props:
Sometimes, child components need to communicate events or changes back to their parent components. This can be achieved by passing callback functions from the parent to the child components as props. The child components then invoke these callback functions to communicate with their parents.
Example 1:
function App(){ const [name, setName] = React.useState("Matt"); return <div> <AppChild name={name} onChangeName={()=>{setName("John")}}/> </div> }
Example 2:
function AppChild(props){ return <span> My name is {props.name} <button onClick={props.onChangeName}>Change Name</button> </span> } ReactDOM.render(<App />, document.getElementById('app'));
In this example, the ChildComponent invokes the onChangeName callback function passed down from the ParentComponent when the button is clicked.
3. Sending Arguments to Callback Props:
Child components often need to send additional data or arguments along with events to their parent components. This can be achieved by passing arguments to callback functions as needed.
Example:
function App(){ const [name, setName] = React.useState("Matt"); //test return <div> <AppChild name={name} onChangeName={(newName)=>{setName(newName)}}/> </div> } function AppChild(props){ return <span> My name is {props.name} <button onClick={()=>props.onChangeName("Bill")}>Change Name</button> </span> } ReactDOM.render(<App />, document.getElementById('app'));
In this example, the ChildComponent sends the argument “Bill” along with the event to the ParentComponent when the button is clicked.
What are ReactJS Lifecycle Methods?
ReactJS lifecycle methods are functions provided by the React library that allow developers to hook into specific points in a component’s lifecycle. These methods enable developers to perform certain actions or execute code at different stages of a component’s existence. Understanding ReactJS lifecycle methods is crucial for building robust and efficient React applications. Let’s delve into the details of these lifecycle methods:
- Initialization: This is the stage where the component is initialized with the given props and default state. In class-based components, initialization typically occurs within the constructor method.
- Mounting: Mounting is the process of rendering the component and inserting it into the DOM. During this stage, the component is created and added to the DOM tree. The componentDidMount() method is called after the component has been mounted.
- Updating: The updating stage occurs when the component’s state or props change, triggering a re-render. React re-renders the component and updates the DOM accordingly. Lifecycle methods such as shouldComponentUpdate(), componentDidUpdate(), and getDerivedStateFromProps() are invoked during this stage.
- Unmounting: Unmounting is the final stage in the component’s lifecycle, where the component is removed from the DOM. The componentWillUnmount() method is called just before the component is unmounted, allowing developers to perform cleanup tasks or unsubscribe from event listeners.
ReactJS Lifecycle Hooks
ReactJS introduced Hooks in version 16.8, providing a new way to manage state and side effects in functional components without the need for class-based components. Let’s explore ReactJS lifecycle hooks in detail:
‒State Hook: The useState hook allows functional components to manage state. It returns a pair of values: the current state and a function to update that state.
‒Effect Hook: The useEffect hook enables functional components to perform side effects, such as fetching data, subscribing to events, or updating the document title. It replaces the functionality of lifecycle methods like componentDidMount, componentDidUpdate, and componentWillUnmount.
React will run this effect after flushing changes to the DOM, ensuring that the document title reflects the current count.
Is React Worth Learning?
React has emerged as the most popular web framework globally, making it highly valuable for developers. Its widespread adoption by enterprises is attributed to its ease of use and powerful features.
React developers command higher salaries compared to other IT specialists due to the growing demand for their expertise. The increasing need for React among businesses worldwide creates opportunities for skilled individuals.
According to data from Indeed, React developers in the USA earn an average salary ranging from 55,000 to 110,000 USD annually. This indicates the high demand for React skills in the job market.
Industry Trends
React is the preferred choice for developers worldwide, surpassing other frameworks and libraries in popularity.
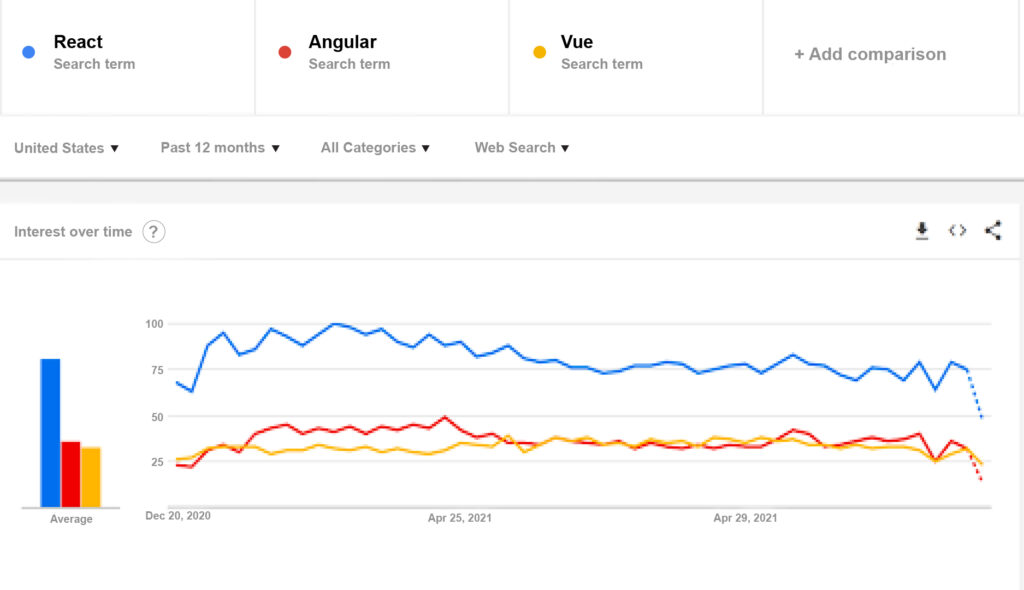
The average salary for entry-level React developers in the USA is approximately 87,000 USD per year, highlighting the lucrative career prospects in this field.
Can a Beginner Start with ReactJS?
Yes, a beginner can start with ReactJS, provided they have a basic understanding of JavaScript, CSS, and HTML, as well as familiarity with ES6 features like arrow functions, template literals, and destructuring assignments. ReactJS is well-documented and has a large community, making it accessible to beginners.
What do I Need to Know to Start Learning ReactJS?
Before diving into ReactJS, it’s essential to have a solid understanding of HTML, CSS, and JavaScript fundamentals.
Interactive Checklist:
Let’s see how prepared you are to start learning ReactJS. Tick the boxes for each skill you feel confident about:
Additionally, familiarity with ES6 features like arrow functions, classes, imports, and exports is beneficial.
How do I Start Learning ReactJS?
Getting started with ReactJS is made easy by its extensive developer community, offering a wealth of resources for learners. Whether you’re interested in compiling a React application, importing images, or running a project locally, there are ample materials available to suit your learning style.
Before diving in, it’s essential to identify your preferred learning method.
Are you inclined towards tutorials or do you prefer video tutorials? Do you thrive on interactive projects or do you prefer mastering theory before practical application?
Answering these questions upfront will streamline your search for learning resources.
Two recommended starting points are W3Schools and the official React website. Both platforms offer comprehensive resources and tutorials tailored to learners at all levels
Conclusion
ReactJS presents an exciting opportunity for both beginners and experienced developers to enhance their skill set and build dynamic, high-performing web applications. With its robust developer community, extensive resources, and intuitive learning curve, getting started with ReactJS is accessible to all. By understanding the basics, exploring its key features, and leveraging its lifecycle methods and hooks, developers can create engaging user interfaces and stay ahead in the rapidly evolving landscape of web development. Whether you’re a seasoned professional or just starting your journey, ReactJS offers a pathway to success and opens doors to lucrative career opportunities in the tech industry.